Deep development of Gimbal with C
There are three approaches to develop for reCamera Gimbal:
- Application Logic Development using Node-RED nodes
- Bash scripting using can-utils tools via terminal commands
- C programming requiring cross-compilation environment setup and binary compilation
This document will provide a comprehensive guide on how to develop for reCamera Gimbal using C programming.
For Node-RED based development, please refer to:
Node-RED Development Documentation
Before developing, you need to install the cross-compile environment into your development environment.
Linux:
sudo apt-get update
sudo apt-get install can-utils
mkdir recamera && cd recamera
wget https://github.com/Seeed-Studio/reCamera-OS/releases/download/0.2.0/reCameraOS_sdk_v0.2.0.tar.gz
tar -xzvf reCameraOS_sdk_v0.2.0.tar.gz
git clone https://github.com/sophgo/host-tools.git
git clone https://github.com/Seeed-Studio/sscma-example-sg200x.git
export SG200X_SDK_PATH=$HOME/recamera/sg2002_recamera_emmc/
export PATH=$HOME/recamera/host-tools/gcc/riscv64-linux-musl-x86_64/bin:$PATH
Download the case program
sudo apt-get install unzip
wget https://files.seeedstudio.com/wiki/reCamera/Gimbal/CAN.zip
unzip CAN.zip
The directory structure is shown as follows:
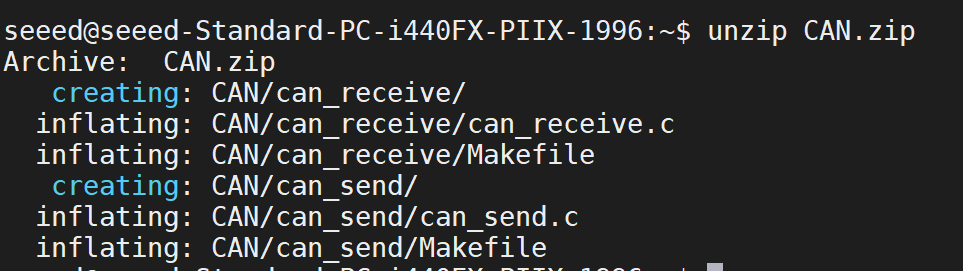
You need to modify the Makefile to replace the CC variable with your own cross-compilation toolchain.
CC = $HOME/recamera/host-tools/gcc/riscv64-linux-musl-x86_64/bin/riscv64-unknown-linux-musl-gcc
Compile the program
cd CAN/can_send
mkdir build && cd build
cmake ../
make
Upload the compiled binary to reCamera
scp can_send recamera@ip_address:/home/recamera
Use candump can0
to view CAN bus data
Use sudo ./can_send
to test the script
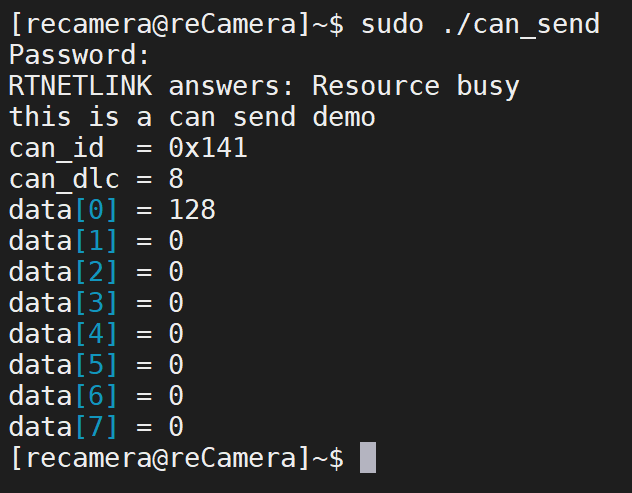
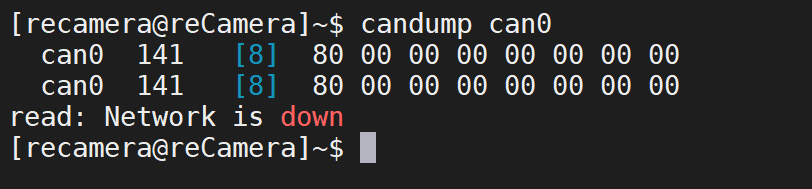
Program Analysis
These commands are used to set the CAN bus rate and interface status, which only need to be initialized once in the application.
"sudo ip link set can0 type can bitrate 100000"
"sudo ifconfig can0 up"
As shown, in the can_send.c file, the above commands are called using the system function.
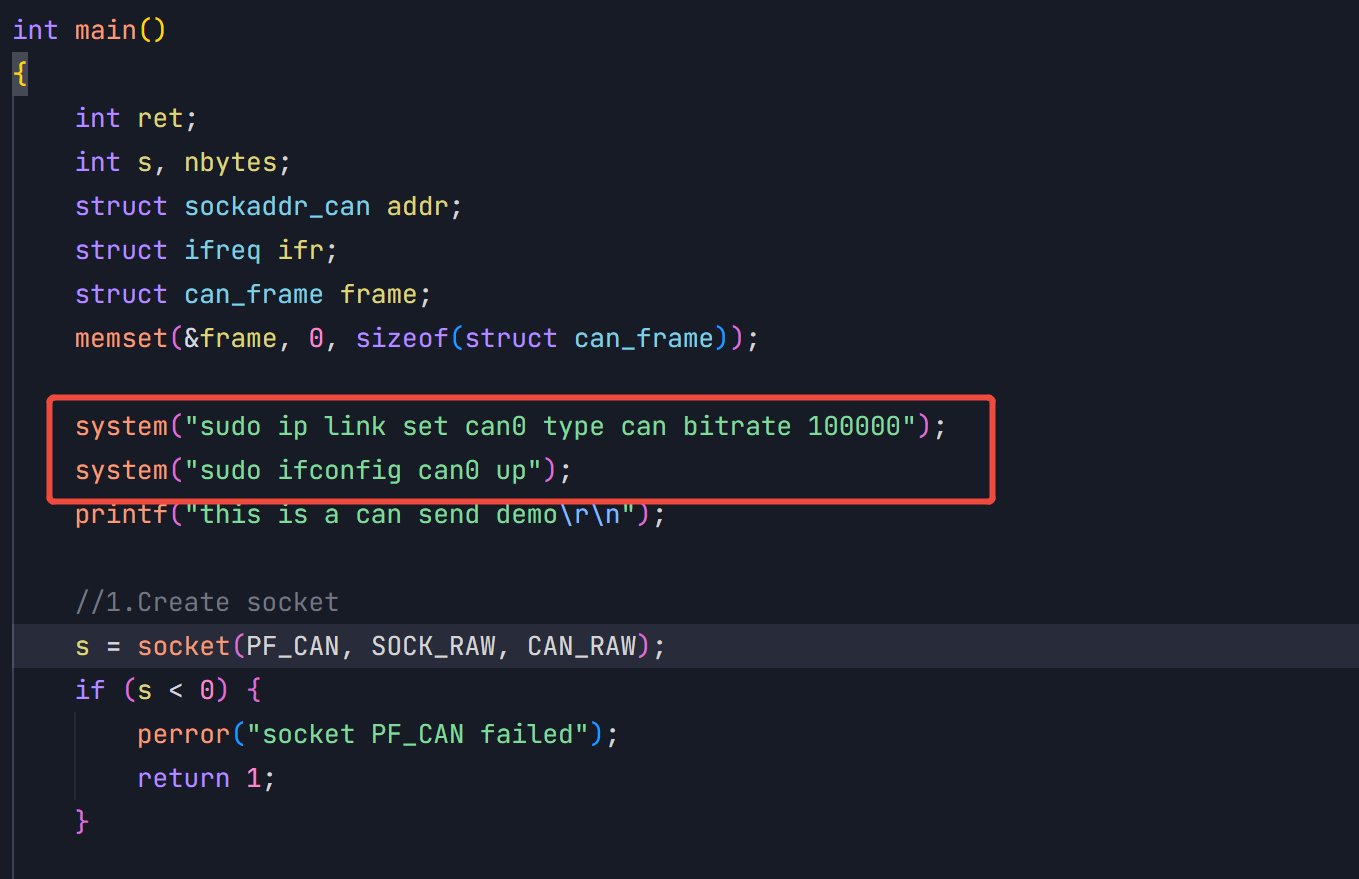
You need to define a CAN frame structure to store the CAN frame's ID, data length, and data. And use the memset function to clear the CAN frame structure to zero.
struct can_frame frame;
memset(&frame, 0, sizeof(struct can_frame));
You can set can_id and can_dlc to configure the CAN frame's ID and data length.
This is the standard CAN frame data format.
Using nbytes = write(s, &frame, sizeof(frame));
to send the CAN frame to the CAN bus, it returns the number of bytes sent. You can compare the returned value with the expected number of bytes to determine if the CAN frame was sent successfully.
//5.Set send data
frame.can_id = 0x141;
frame.can_dlc = 8;
frame.data[0] = 0x80;
frame.data[1] = 0x00;
frame.data[2] = 0x00;
frame.data[3] = 0x00;
frame.data[4] = 0x00;
frame.data[5] = 0x00;;
frame.data[6] = 0x00;
frame.data[7] = 0x00
You can refer to more C programs in our Github repository.
Please note that the Gimbal-related programs are developed based on Node-RED components. We will only maintain Node-RED programs going forward, so you can directly reference and develop in Node-RED.
Since the C programs are not maintained, they may become invalid as the motor firmware updates. Please use our latest motor manual to encapsulate the correct commands for development.
Resources
Tech Support & Product Discussion
Thank you for choosing our products! We are here to provide you with different support to ensure that your experience with our products is as smooth as possible. We offer several communication channels to cater to different preferences and needs.