Using XIAO ESP32S3 Sense as an AI Sensor with SenseCraft AI
This wiki provides a step-by-step guide on how to configure the output of a model on SenseCraft AI and use the XIAO ESP32S3 Sense as an AI sensor. By following these instructions, you will learn how to connect the XIAO ESP32S3 Sense to your computer, select the appropriate output method, and retrieve the model data using different communication protocols such as UART, I2C, and SPI.
Prerequisites
Before proceeding, ensure that you have the following:
- XIAO ESP32S3 Sense
- An additional XIAO board (e.g., XIAO ESP32C3) for receiving the model data
- USB-C data cable for connecting the XIAO ESP32S3 Sense to your computer
- Arduino IDE with the Seeed_Arduino_SSCMA library installed
XIAO ESP32S3 Sense |
---|
![]() |
Step 1. Access the SenseCraft AI Vision Workspace and connect the XIAO ESP32S3 Sense
Open your web browser and navigate to the SenseCraft AI Vision Workspace page.
Select the XIAO ESP32S3 Sense board from the available devices.
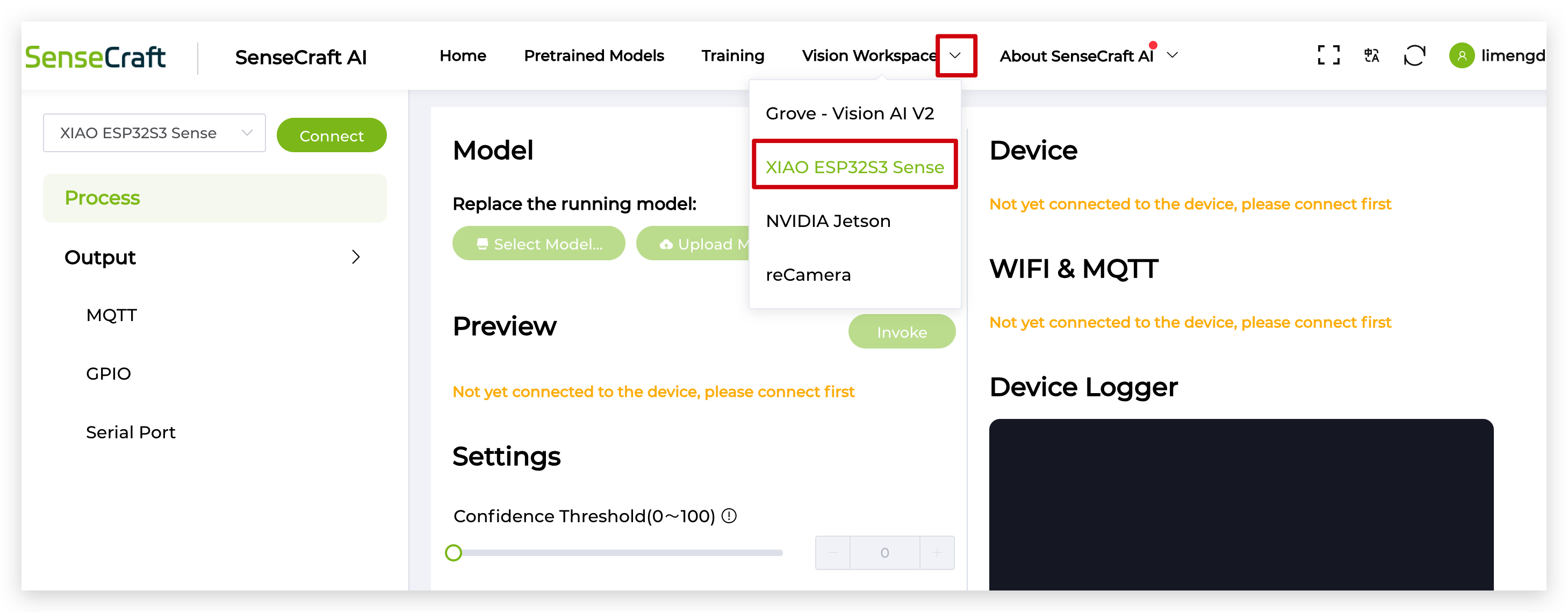
Using the USB-C cable, connect your XIAO ESP32S3 Sense board to your computer. Once connected, click the Connect button located in the top-left corner of the SenseCraft AI Vision Workspace page.
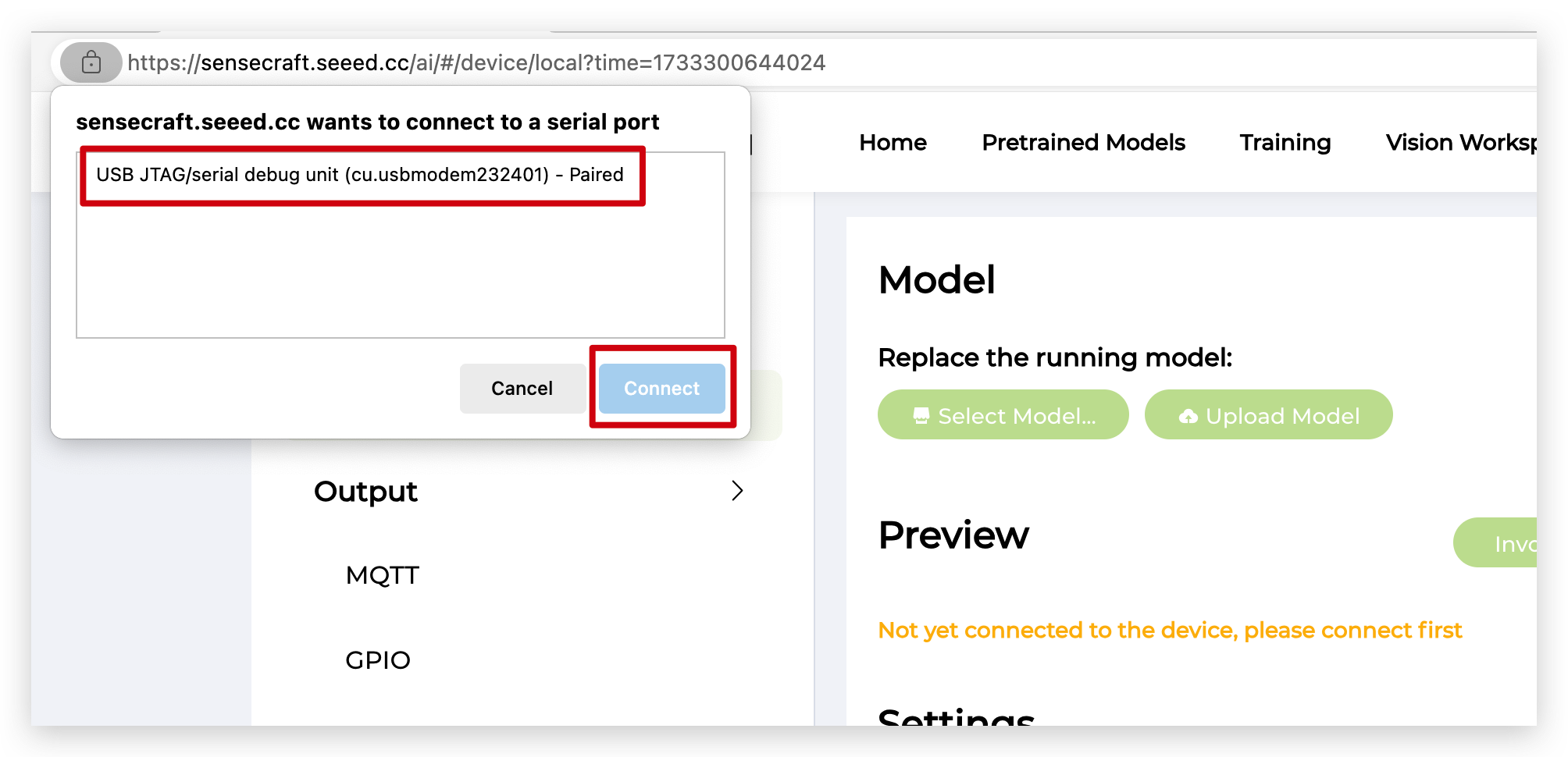
Step 2. Ensure a Model is Loaded on the XIAO ESP32S3 Sense
Before proceeding, make sure that your XIAO ESP32S3 Sense board has a trained model loaded. If you haven't loaded a model yet, refer to the SenseCraft AI documentation on how to train and deploy models to your device.
If you want to use your own trained model, you can refer to the following two Wikis.
Step 3. Prepare the way and program you want to use
XIAO supports outputting model results via UART, IIC or SPI, you can choose how you want to output the results according to the actual situation.
Option 1. UART Communication
Connect the TX and RX pins of the two XIAO boards together. For the AI Sensor (already modeled) XIAO ESP32S3 Sense, the output pins are defined as:
- TX: GPIO43
- RX: GPIO44
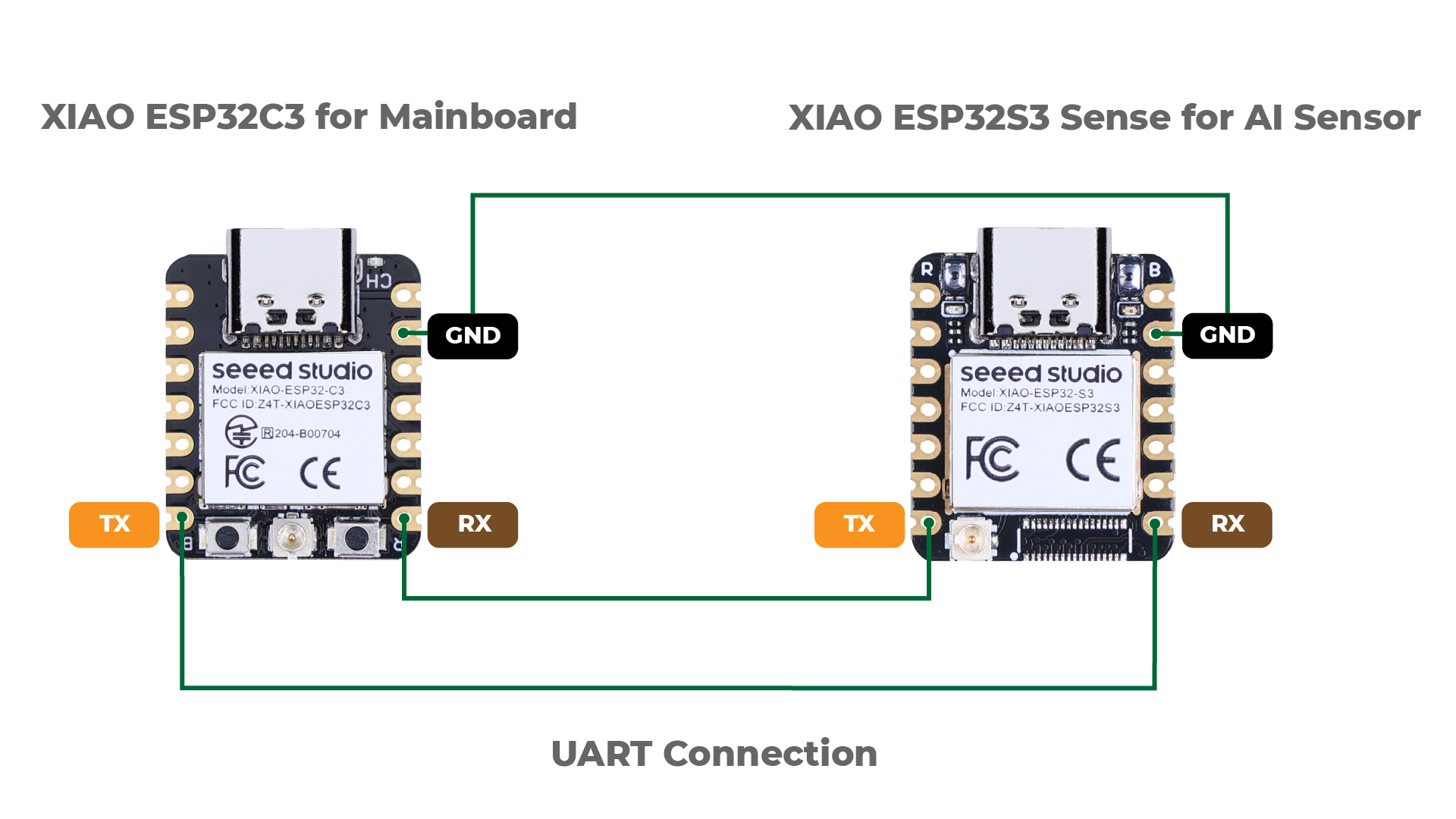
Download the Seeed_Arduino_SSCMA library from GitHub and add it to your Arduino environment.
Use the code from the following example:
Set the baud rate to 921600 with 8 data bits, no parity, and 1 stop bit (8N1).
#include <Seeed_Arduino_SSCMA.h>
#ifdef ESP32
#include <HardwareSerial.h>
// Define two Serial devices mapped to the two internal UARTs
HardwareSerial atSerial(0);
#else
#define atSerial Serial1
#endif
SSCMA AI;
void setup()
{
Serial.begin(9600);
AI.begin(&atSerial);
}
void loop()
{
if (!AI.invoke(1,false,true))
{
Serial.println("invoke success");
Serial.print("perf: prepocess=");
Serial.print(AI.perf().prepocess);
Serial.print(", inference=");
Serial.print(AI.perf().inference);
Serial.print(", postpocess=");
Serial.println(AI.perf().postprocess);
for (int i = 0; i < AI.boxes().size(); i++)
{
Serial.print("Box[");
Serial.print(i);
Serial.print("] target=");
Serial.print(AI.boxes()[i].target);
Serial.print(", score=");
Serial.print(AI.boxes()[i].score);
Serial.print(", x=");
Serial.print(AI.boxes()[i].x);
Serial.print(", y=");
Serial.print(AI.boxes()[i].y);
Serial.print(", w=");
Serial.print(AI.boxes()[i].w);
Serial.print(", h=");
Serial.println(AI.boxes()[i].h);
}
for (int i = 0; i < AI.classes().size(); i++)
{
Serial.print("Class[");
Serial.print(i);
Serial.print("] target=");
Serial.print(AI.classes()[i].target);
Serial.print(", score=");
Serial.println(AI.classes()[i].score);
}
for (int i = 0; i < AI.points().size(); i++)
{
Serial.print("Point[");
Serial.print(i);
Serial.print("]: target=");
Serial.print(AI.points()[i].target);
Serial.print(", score=");
Serial.print(AI.points()[i].score);
Serial.print(", x=");
Serial.print(AI.points()[i].x);
Serial.print(", y=");
Serial.println(AI.points()[i].y);
}
for (int i = 0; i < AI.keypoints().size(); i++)
{
Serial.print("keypoint[");
Serial.print(i);
Serial.print("] target=");
Serial.print(AI.keypoints()[i].box.target);
Serial.print(", score=");
Serial.print(AI.keypoints()[i].box.score);
Serial.print(", box:[x=");
Serial.print(AI.keypoints()[i].box.x);
Serial.print(", y=");
Serial.print(AI.keypoints()[i].box.y);
Serial.print(", w=");
Serial.print(AI.keypoints()[i].box.w);
Serial.print(", h=");
Serial.print(AI.keypoints()[i].box.h);
Serial.print("], points:[");
for (int j = 0; j < AI.keypoints()[i].points.size(); j++)
{
Serial.print("[");
Serial.print(AI.keypoints()[i].points[j].x);
Serial.print(",");
Serial.print(AI.keypoints()[i].points[j].y);
Serial.print("],");
}
Serial.println("]");
}
if(!AI.last_image().isEmpty())
{
Serial.print("Last image:");
Serial.println(AI.last_image().c_str());
}
}
}
If all goes well, you will see the output message of the model result as shown below.
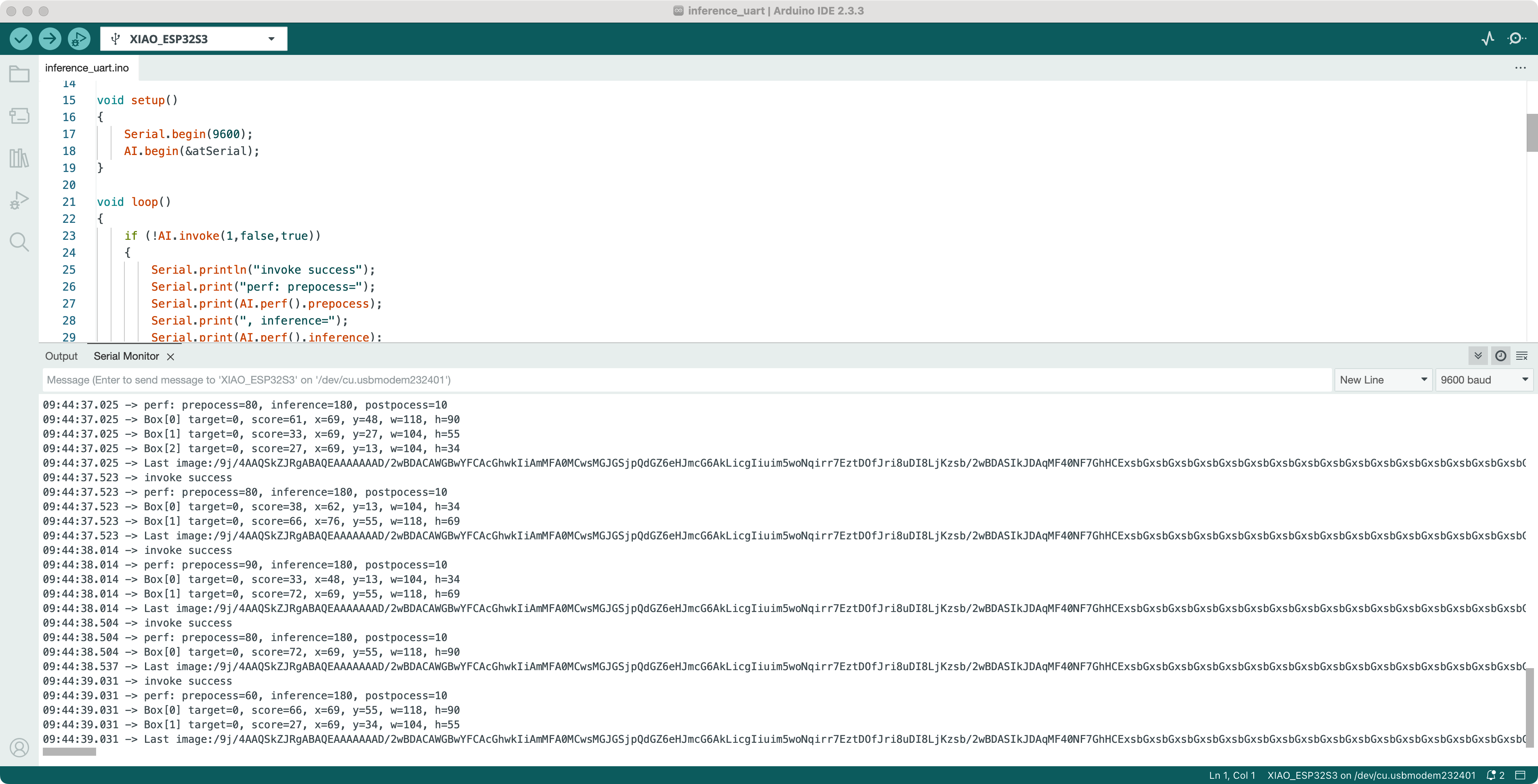
Option 2. I2C Communication
Connect the SDA and SCL pins of the two XIAO boards together. For the AI Sensor (already modeled) XIAO ESP32S3 Sense, the output pins are defined as:
- SDA: GPIO5 (with pull-up resistor)
- SCL: GPIO6 (with pull-up resistor)
- I2C slave address to
0x62
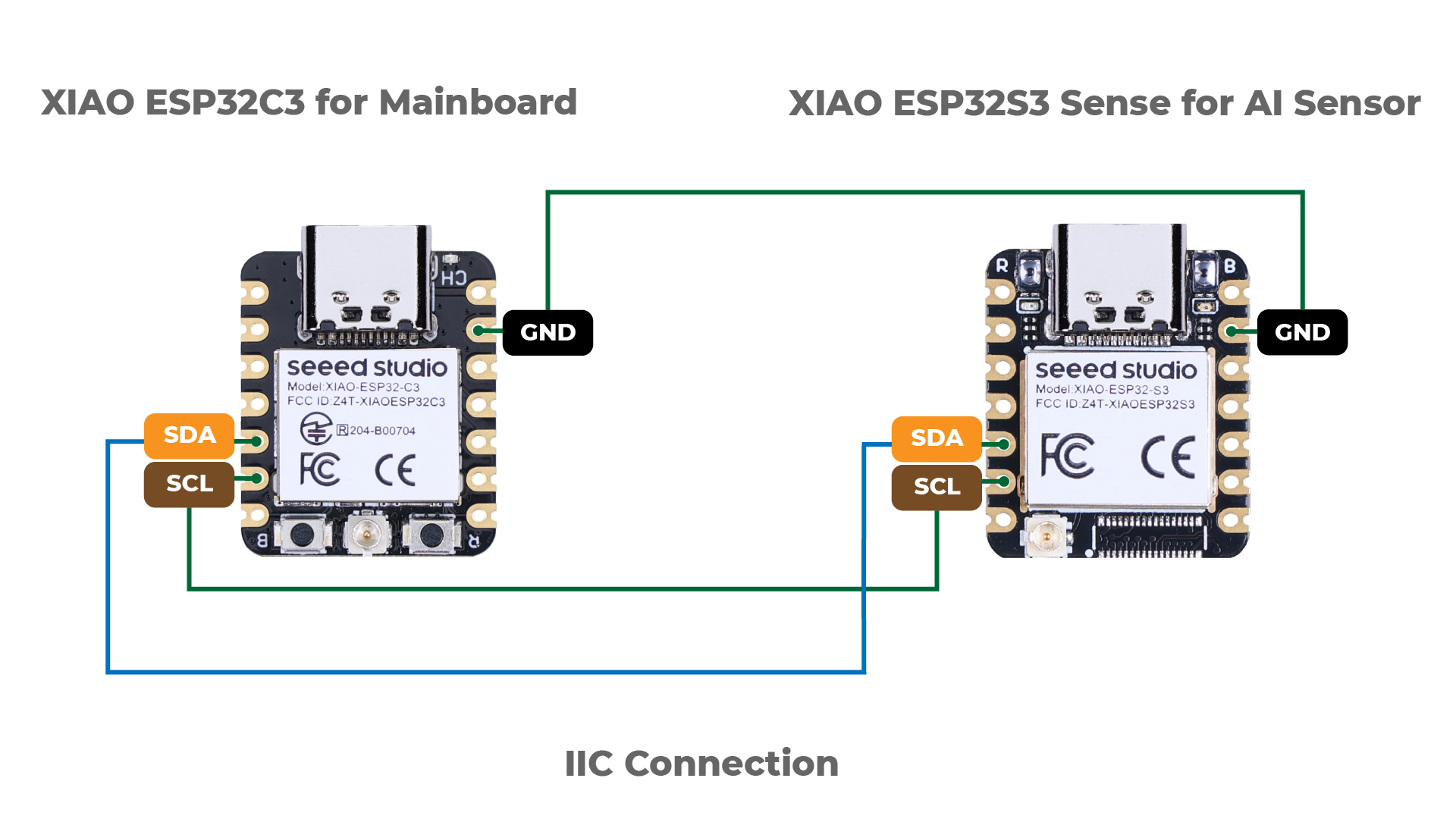
Download the Seeed_Arduino_SSCMA library from GitHub and add it to your Arduino environment.
Use the code from the following example:
Use the following protocol for I2C communication:
- READ: Send bytes 0x10, 0x01, LEN << 8, LEN & 0xff
- WRITE: Send bytes 0x10, 0x02, LEN << 8, LEN & 0xff
- AVAIL (to check data availability): Send bytes 0x10, 0x03
- RESET (to clear buffer): Send bytes 0x10, 0x06
#include <Seeed_Arduino_SSCMA.h>
#include <Wire.h>
SSCMA AI;
void setup()
{
Wire.begin();
AI.begin(&Wire);
Serial.begin(9600);
}
void loop()
{
if (!AI.invoke())
{
Serial.println("invoke success");
Serial.print("perf: prepocess=");
Serial.print(AI.perf().prepocess);
Serial.print(", inference=");
Serial.print(AI.perf().inference);
Serial.print(", postpocess=");
Serial.println(AI.perf().postprocess);
for (int i = 0; i < AI.boxes().size(); i++)
{
Serial.print("Box[");
Serial.print(i);
Serial.print("] target=");
Serial.print(AI.boxes()[i].target);
Serial.print(", score=");
Serial.print(AI.boxes()[i].score);
Serial.print(", x=");
Serial.print(AI.boxes()[i].x);
Serial.print(", y=");
Serial.print(AI.boxes()[i].y);
Serial.print(", w=");
Serial.print(AI.boxes()[i].w);
Serial.print(", h=");
Serial.println(AI.boxes()[i].h);
}
for (int i = 0; i < AI.classes().size(); i++)
{
Serial.print("Class[");
Serial.print(i);
Serial.print("] target=");
Serial.print(AI.classes()[i].target);
Serial.print(", score=");
Serial.println(AI.classes()[i].score);
}
for (int i = 0; i < AI.points().size(); i++)
{
Serial.print("Point[");
Serial.print(i);
Serial.print("]: target=");
Serial.print(AI.points()[i].target);
Serial.print(", score=");
Serial.print(AI.points()[i].score);
Serial.print(", x=");
Serial.print(AI.points()[i].x);
Serial.print(", y=");
Serial.println(AI.points()[i].y);
}
for (int i = 0; i < AI.keypoints().size(); i++)
{
Serial.print("keypoint[");
Serial.print(i);
Serial.print("] target=");
Serial.print(AI.keypoints()[i].box.target);
Serial.print(", score=");
Serial.print(AI.keypoints()[i].box.score);
Serial.print(", box:[x=");
Serial.print(AI.keypoints()[i].box.x);
Serial.print(", y=");
Serial.print(AI.keypoints()[i].box.y);
Serial.print(", w=");
Serial.print(AI.keypoints()[i].box.w);
Serial.print(", h=");
Serial.print(AI.keypoints()[i].box.h);
Serial.print("], points:[");
for (int j = 0; j < AI.keypoints()[i].points.size(); j++)
{
Serial.print("[");
Serial.print(AI.keypoints()[i].points[j].x);
Serial.print(",");
Serial.print(AI.keypoints()[i].points[j].y);
Serial.print("],");
}
Serial.println("]");
}
}
}
If all goes well, you will see the output message of the model result as shown below.
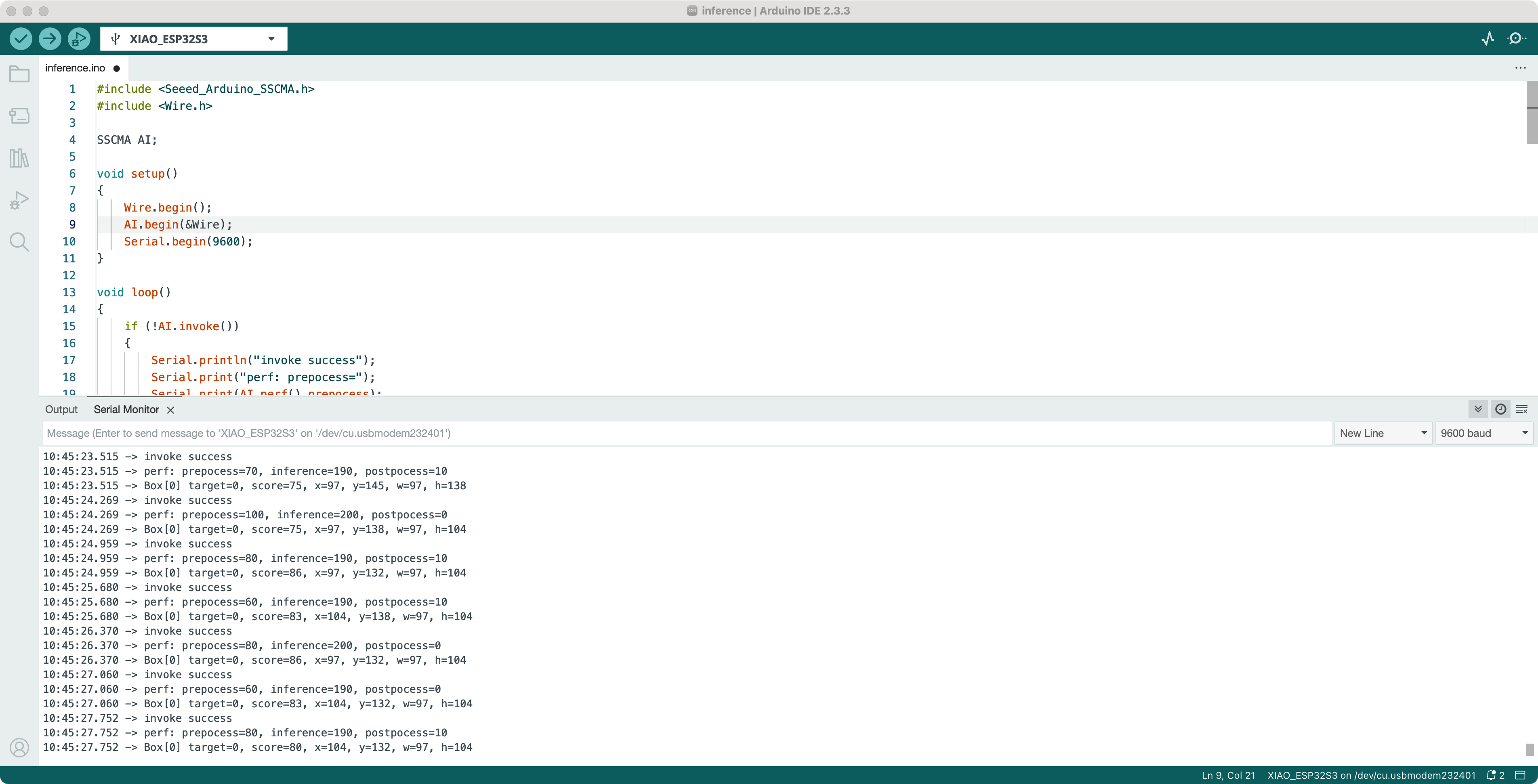
Option 3. SPI Communication
- Connect the following pins of the two XIAO boards together. For the AI Sensor (already modeled) XIAO ESP32S3 Sense, the output pins are defined as:
- MOSI: GPIO9 (with pull-up resistor)
- MISO: GPIO8
- SCLK: GPIO7 (with pull-up resistor)
- CS: GPIO4 (with pull-up resistor)
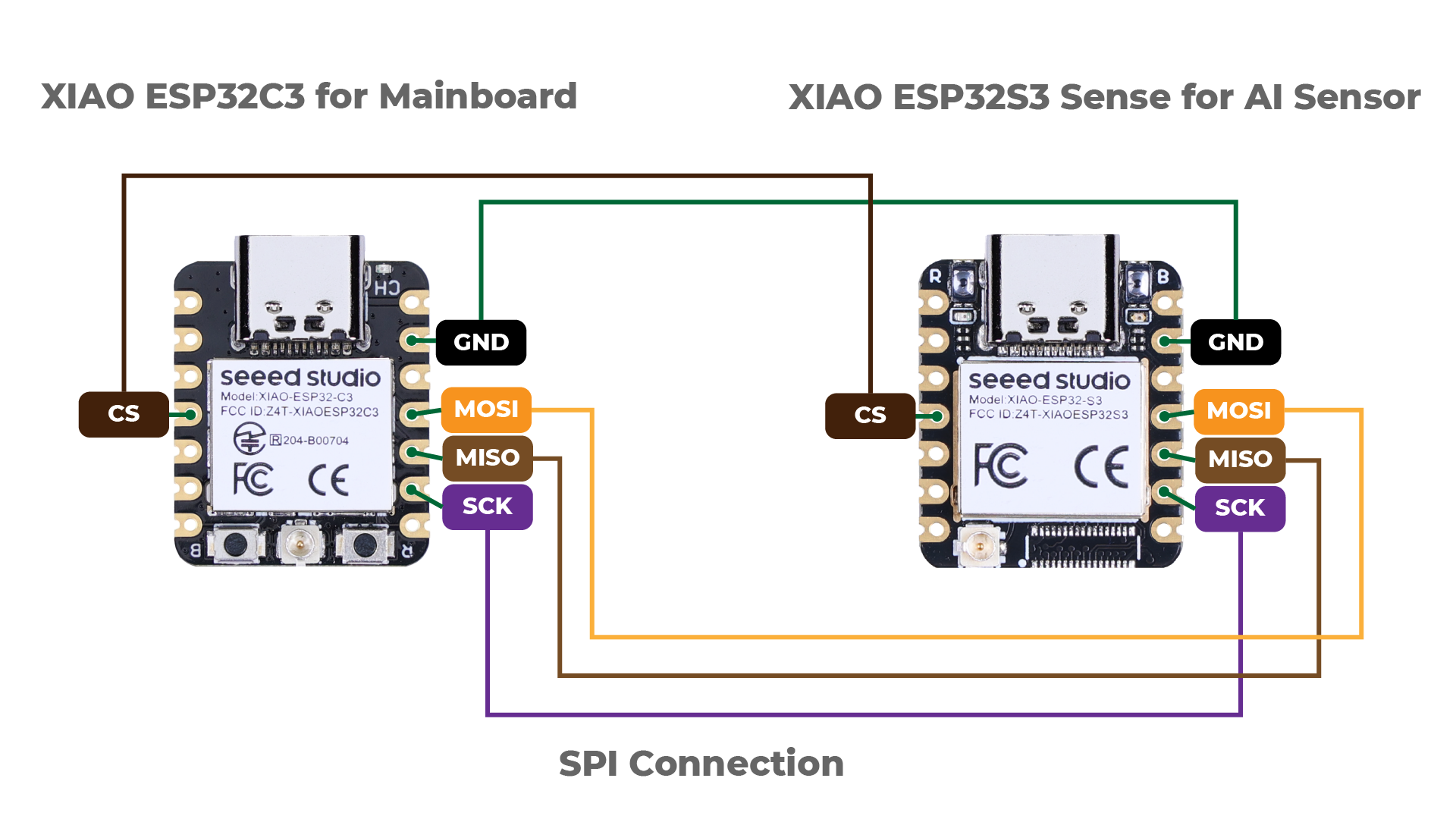
- Download the Seeed_Arduino_SSCMA library from GitHub and add it to your Arduino environment.
- Modify the code from the provided examples to use SPI communication.
Use the following protocol for SPI communication:
- READ: Send bytes 0x10, 0x01, LEN << 8, LEN & 0xff
- WRITE: Send bytes 0x10, 0x02, LEN << 8, LEN & 0xff
- AVAIL (to check data availability): Send bytes 0x10, 0x03
- RESET (to clear buffer): Send bytes 0x10, 0x06
#include <Seeed_Arduino_SSCMA.h>
#include <SPI.h>
SSCMA AI;
void setup()
{
SPI.begin(SCK, MISO, MOSI, -1);
AI.begin(&SPI, D3, -1, -1);
Serial.begin(9600);
}
void loop()
{
if (!AI.invoke())
{
Serial.println("invoke success");
Serial.print("perf: prepocess=");
Serial.print(AI.perf().prepocess);
Serial.print(", inference=");
Serial.print(AI.perf().inference);
Serial.print(", postpocess=");
Serial.println(AI.perf().postprocess);
for (int i = 0; i < AI.boxes().size(); i++)
{
Serial.print("Box[");
Serial.print(i);
Serial.print("] target=");
Serial.print(AI.boxes()[i].target);
Serial.print(", score=");
Serial.print(AI.boxes()[i].score);
Serial.print(", x=");
Serial.print(AI.boxes()[i].x);
Serial.print(", y=");
Serial.print(AI.boxes()[i].y);
Serial.print(", w=");
Serial.print(AI.boxes()[i].w);
Serial.print(", h=");
Serial.println(AI.boxes()[i].h);
}
for (int i = 0; i < AI.classes().size(); i++)
{
Serial.print("Class[");
Serial.print(i);
Serial.print("] target=");
Serial.print(AI.classes()[i].target);
Serial.print(", score=");
Serial.println(AI.classes()[i].score);
}
for (int i = 0; i < AI.points().size(); i++)
{
Serial.print("Point[");
Serial.print(i);
Serial.print("]: target=");
Serial.print(AI.points()[i].target);
Serial.print(", score=");
Serial.print(AI.points()[i].score);
Serial.print(", x=");
Serial.print(AI.points()[i].x);
Serial.print(", y=");
Serial.println(AI.points()[i].y);
}
for (int i = 0; i < AI.keypoints().size(); i++)
{
Serial.print("keypoint[");
Serial.print(i);
Serial.print("] target=");
Serial.print(AI.keypoints()[i].box.target);
Serial.print(", score=");
Serial.print(AI.keypoints()[i].box.score);
Serial.print(", box:[x=");
Serial.print(AI.keypoints()[i].box.x);
Serial.print(", y=");
Serial.print(AI.keypoints()[i].box.y);
Serial.print(", w=");
Serial.print(AI.keypoints()[i].box.w);
Serial.print(", h=");
Serial.print(AI.keypoints()[i].box.h);
Serial.print("], points:[");
for (int j = 0; j < AI.keypoints()[i].points.size(); j++)
{
Serial.print("[");
Serial.print(AI.keypoints()[i].points[j].x);
Serial.print(",");
Serial.print(AI.keypoints()[i].points[j].y);
Serial.print("],");
}
Serial.println("]");
}
}
}
If all goes well, you will see the output message of the model result as shown below.
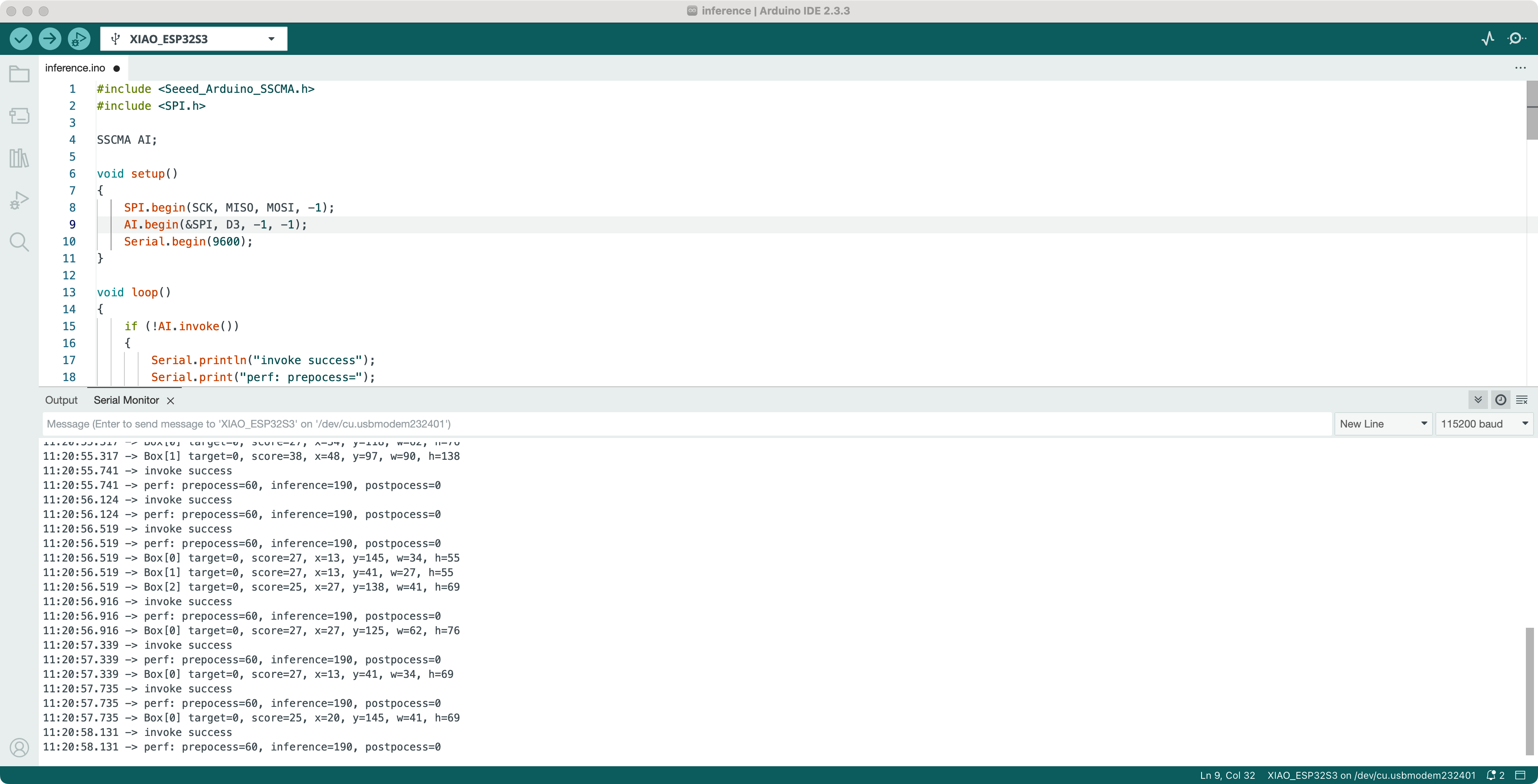
Step 4: Upload the Code and Run
Open the appropriate example code in the Arduino IDE based on your chosen communication protocol. Verify and upload the code to the receiving XIAO board. Open the serial monitor in the Arduino IDE to view the received model data.
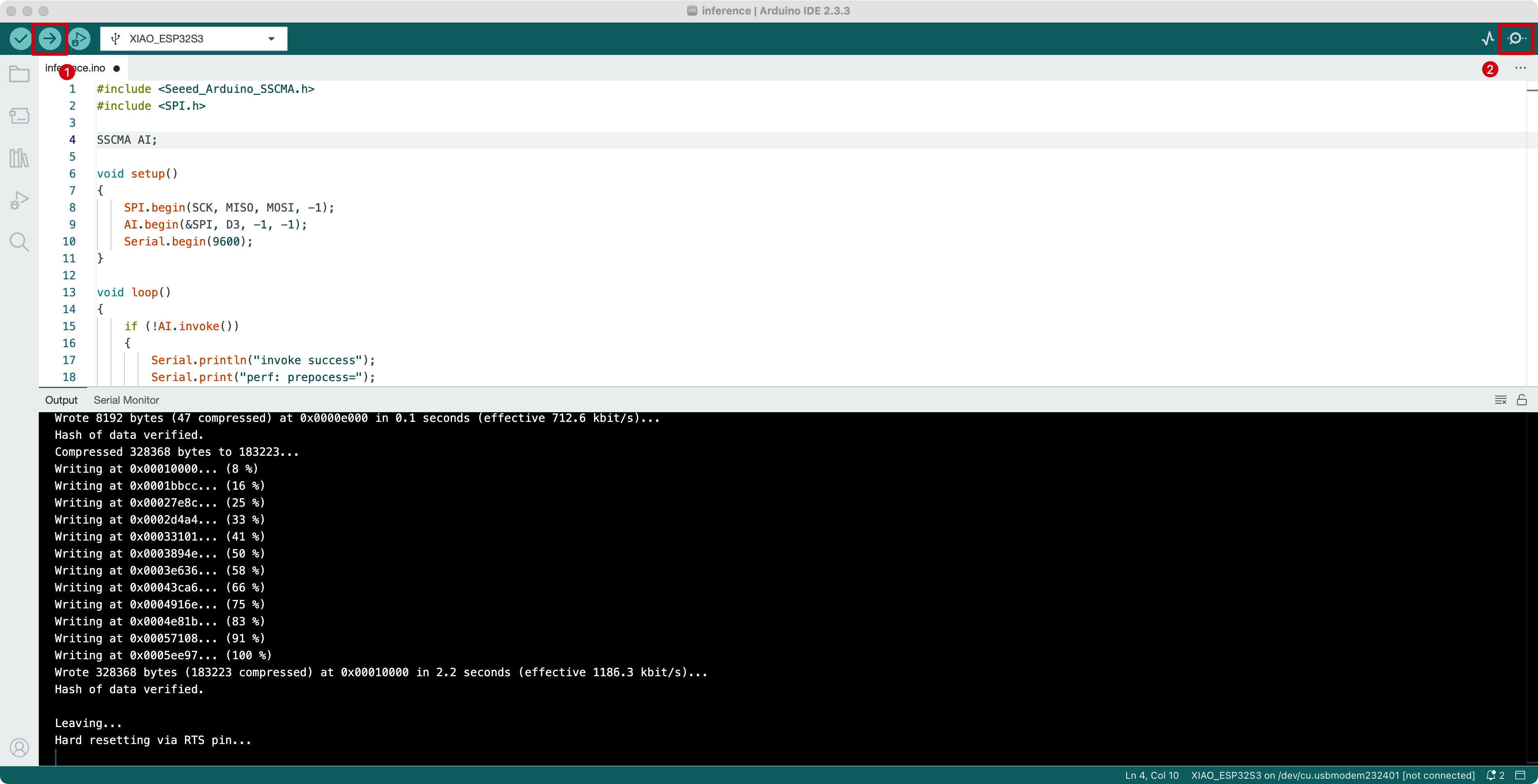
Conclusion
By following this step-by-step guide, you have successfully configured the output of a model on SenseCraft AI and used the XIAO ESP32S3 Sense as an AI sensor. You can now retrieve the model data using different communication protocols such as UART, I2C, or SPI, depending on your project requirements.
Remember to refer to the provided example code and make necessary modifications based on your specific setup and communication protocol choice.
If you encounter any issues or have further questions, please consult the Seeed Studio documentation or seek assistance from the community forums.
Happy sensing with your XIAO ESP32S3 Sense and SenseCraft AI!
Tech Support & Product Discussion
Thank you for choosing our products! We are here to provide you with different support to ensure that your experience with our products is as smooth as possible. We offer several communication channels to cater to different preferences and needs.