Grove - DS1307 RTC (Real Time Clock) for Arduino
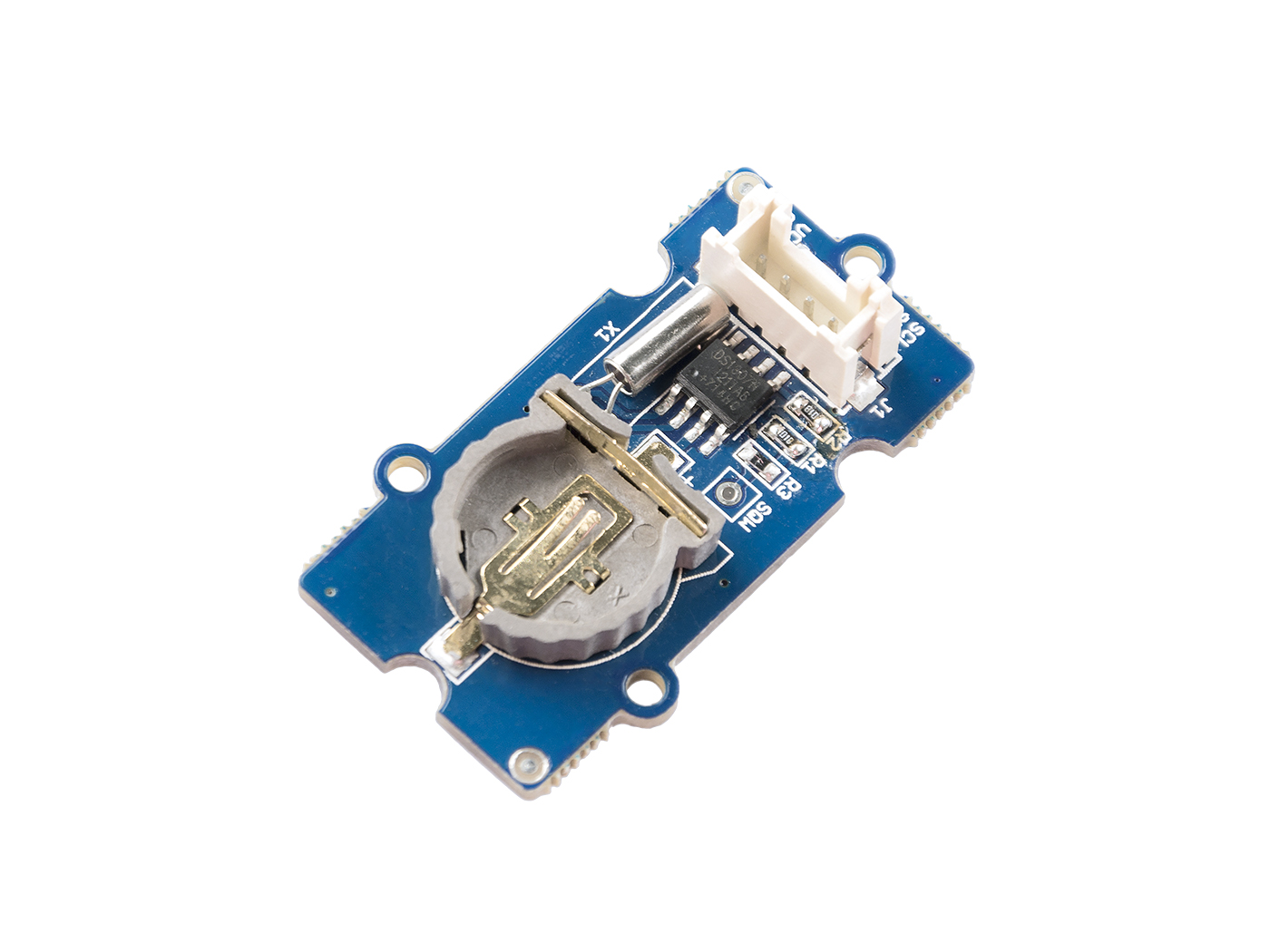
The RTC module is based on the clock chip DS1307, which supports the I2C protocol. It utilizes a Lithium cell battery (CR1225). The clock/calendar provides seconds, minutes, hours, day, date, month, and year. The end of the month date is automatically adjusted for months with fewer than 31 days, including corrections for leap years. The clock operates in either the 24-hour or 12-hour format with AM/PM indicator. And it is valid up to 2100. In order to gain a robust performance, you must put a 3-Volt CR1225 lithium cell in the battery-holder. If you use the primary power only, the module may not work normally, because the crystal may not oscillate.
The battery is not included.
Pre-reading
An introduction of RTC for Arduino is strongly recommended reading ahead if you are not familiar with it:
Specifications
- PCB Size: 2.0cm*4.0cm
- Interface: 2.0mm pitch pin header
- IO Structure: SCL,SDA,VCC,GND
- ROHS: YES
- VCC:3.3~5.5V
- Logic High Level Input :2.2~VCC+0.3 V
- Logic Low Level Input :-0.3~+0.8 V
- Battery Voltage:2.0~3.5 V
More details about Grove modules please refer to Grove System
Platforms Supported
Arduino | Raspberry Pi | |||
---|---|---|---|---|
![]() | ![]() | ![]() | ![]() | ![]() |
The platforms mentioned above as supported is/are an indication of the module's software or theoritical compatibility. We only provide software library or code examples for Arduino platform in most cases. It is not possible to provide software library / demo code for all possible MCU platforms. Hence, users have to write their own software library.
Getting started
Play with Arduino
Hardware
- Step 1. Prepare the below stuffs:
Seeeduino V4.2 | Base Shield | Grove-RTC |
---|---|---|
![]() | ![]() | ![]() |
Get One Now | Get One Now | Get One Now |
- Step 2. Connect Grove-RTC to port I2C of Grove-Base Shield.
- Step 3. Plug Grove - Base Shield into Seeeduino.
- Step 4. Connect Seeeduino to PC via a USB cable.
In order to gain a robust performance, you must put a 3-Volt CR1225 lithium cell in the battery-holder. If you use the primary power only, the module may not work normally, because the crystal may not oscillate.
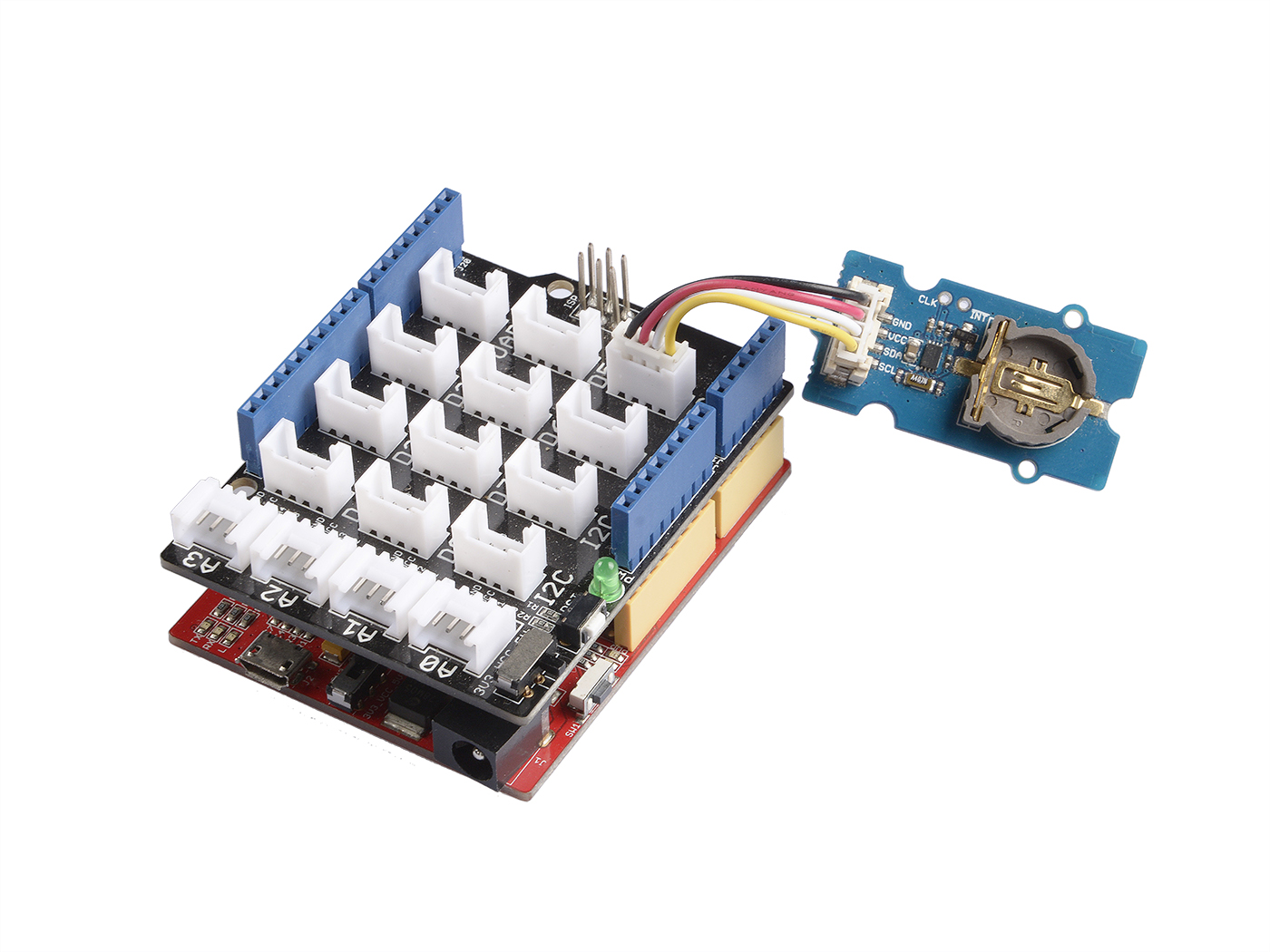
If we don't have the base shield, we also can directly connect the Grove-RTC to Arduino board. Please follow below connection.
Seeeduino_v4 | Grove-RTC |
---|---|
5V | VCC |
GND | GND |
SDA | SDA |
SCL | SCL |
Software
Step 1. Download the RTC Library.
Step 2. Refer How to install library to install library for Arduino.
Step 3. Create a new Arduino sketch and paste the codes below to it or open the code directly by the path: File -> Example ->RTC->SetTimeAndDisplay.
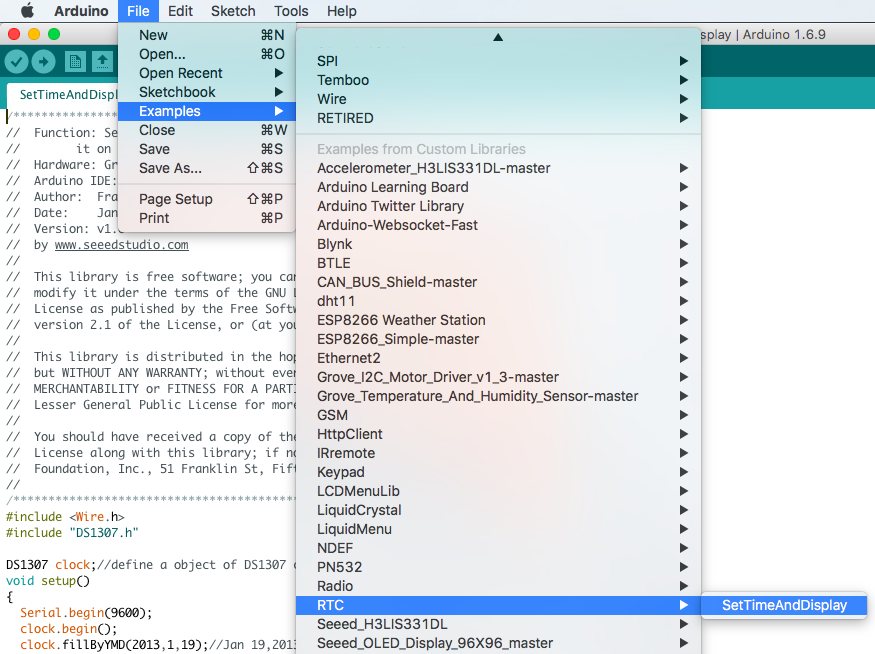
#include <Wire.h>
#include "DS1307.h"
DS1307 clock;//define a object of DS1307 class
void setup()
{
Serial.begin(9600);
clock.begin();
clock.fillByYMD(2013,1,19);//Jan 19,2013
clock.fillByHMS(15,28,30);//15:28 30"
clock.fillDayOfWeek(SAT);//Saturday
clock.setTime();//write time to the RTC chip
}
void loop()
{
printTime();
}
/*Function: Display time on the serial monitor*/
void printTime()
{
clock.getTime();
Serial.print(clock.hour, DEC);
Serial.print(":");
Serial.print(clock.minute, DEC);
Serial.print(":");
Serial.print(clock.second, DEC);
Serial.print(" ");
Serial.print(clock.month, DEC);
Serial.print("/");
Serial.print(clock.dayOfMonth, DEC);
Serial.print("/");
Serial.print(clock.year+2000, DEC);
Serial.print(" ");
Serial.print(clock.dayOfMonth);
Serial.print("*");
switch (clock.dayOfWeek)// Friendly printout the weekday
{
case MON:
Serial.print("MON");
break;
case TUE:
Serial.print("TUE");
break;
case WED:
Serial.print("WED");
break;
case THU:
Serial.print("THU");
break;
case FRI:
Serial.print("FRI");
break;
case SAT:
Serial.print("SAT");
break;
case SUN:
Serial.print("SUN");
break;
}
Serial.println(" ");
}
Step 4. Set the time. Change function arguments to current date/time. Please pay attention to arguments' format.
clock.fillByYMD(2013,1,19);//Jan 19,2013
clock.fillByHMS(15,28,30);//15:28 30"
clock.fillDayOfWeek(SAT);//Saturday
Step 5. Upload the code and open the serial monitor to receive the sensor's data
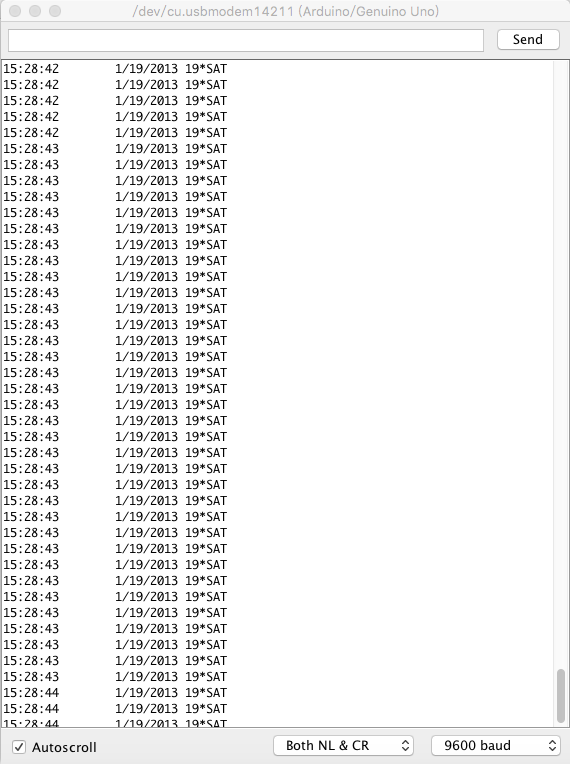
Play With Raspberry Pi
Hardware
- Step 1. Prepare the below stuffs:
Raspberry pi | GrovePi_Plus | Grove-RTC |
---|---|---|
![]() | ![]() | ![]() |
Get One Now | Get One Now | Get One Now |
- Step 2. Plug the GrovePi_Plus into Raspberry.
- Step 3. Connect Grove-RTC to I2C port of GrovePi_Plus.
- Step 4. Connect the Raspberry to PC through USB cable.
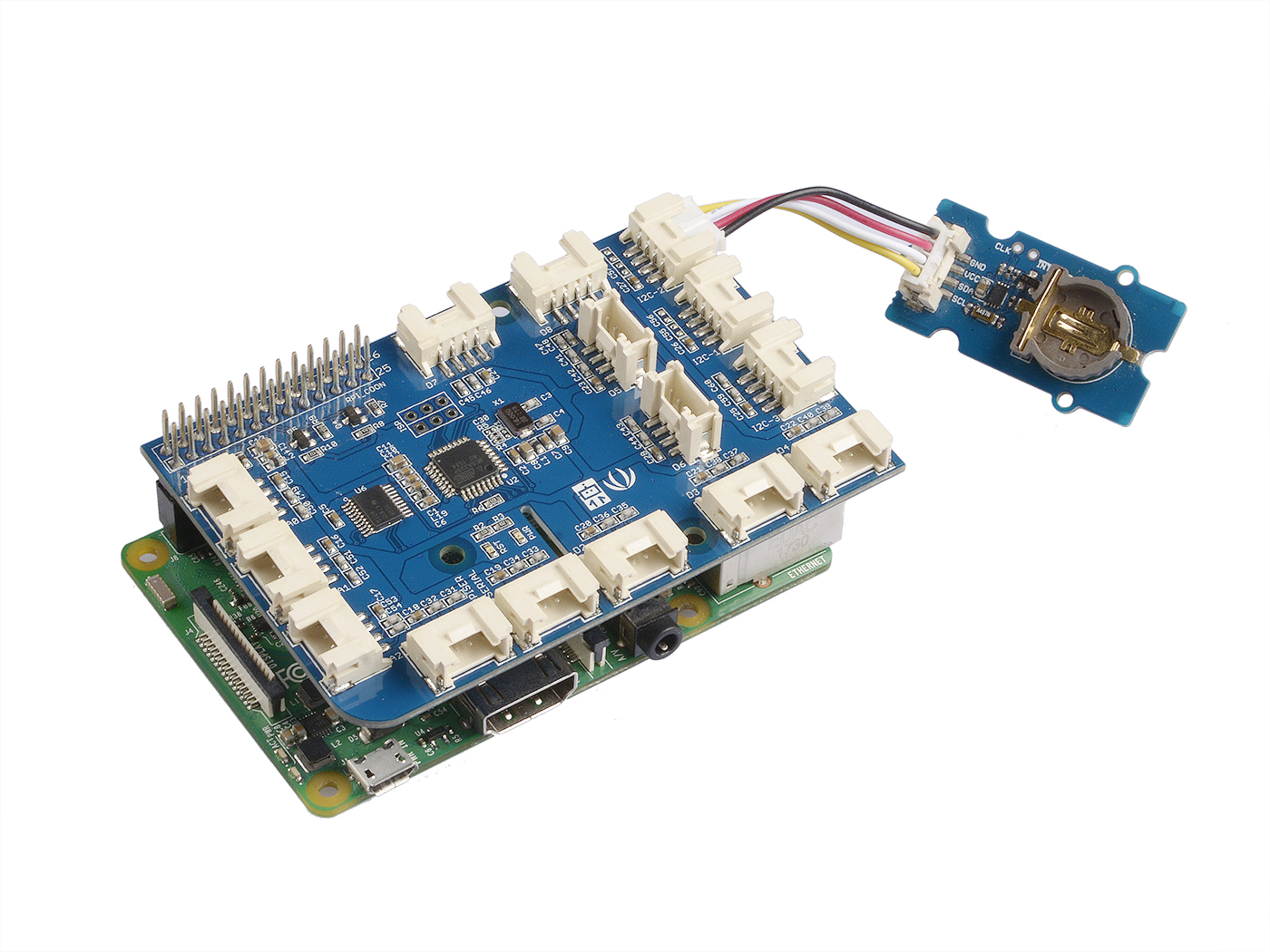
Software
- Step 1. Follow Setting Software to configure the development environment.
- Step 2. Git clone the Github repository.
cd ~
git clone https://github.com/DexterInd/GrovePi.git
- Step 3. Excute below commands to use this sensor
cd ~/GrovePi/Software/Python
python grove_i2c_rtc.py
Here is the code
#!/usr/bin/env python
#
# GrovePi Example for using the Grove I2C RTC (https://www.seeedstudio.com/wiki/Grove_-_RTC)
#
# The GrovePi connects the Raspberry Pi and Grove sensors. You can learn more about GrovePi here: http://www.dexterindustries.com/GrovePi
#
# Have a question about this example? Ask on the forums here: http://forum.dexterindustries.com/c/grovepi
#
'''
## License
The MIT License (MIT)
GrovePi for the Raspberry Pi: an open source platform for connecting Grove Sensors to the Raspberry Pi.
Copyright (C) 2017 Dexter Industries
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files (the "Software"), to deal
in the Software without restriction, including without limitation the rights
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
copies of the Software, and to permit persons to whom the Software is
furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in
all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
THE SOFTWARE.
'''
import time
import grovepi
# Connect the Grove Real Time Clock to any I2C port eg. I2C-1
# Can be found at I2C address 0x68
# SCL,SDA,VCC,GND
while True:
try:
print(grovepi.rtc_getTime())
time.sleep(.5)
except IOError:
print ("Error")
Step 4. Here is the result.
Demo 2: Grove_rtc
You can also use this demo to show the time in common. Please create grove_rtc.py and copy below codes.
'''
* Grove-RTC.py
* Demo for Raspberry Pi
*
* Copyright (c) 2014 seeed technology inc.
* Website : community.seeedstudio.com/
* Author : Lambor
* Create Time: Nov 2014
* Change Log :
*
* The MIT License (MIT)
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
* THE SOFTWARE.
'''
#!/usr/bin/python
import time
import smbus
bus = smbus.SMBus(1) # 0 = /dev/i2c-0 (port I2C0), 1 = /dev/i2c-1 (port I2C1)
class DS1307():
def __init__(self):
self.MON = 1
self.TUE = 2
self.WED = 3
self.THU = 4
self.FRI = 5
self.SAT = 6
self.SUN = 7
self.DS1307_I2C_ADDRESS = 0x68
print 'begin'
def decToBcd(self, val):
return ( (val/10*16) + (val%10) )
def bcdToDec(self, val):
return ( (val/16*10) + (val%16) )
def begin(self, news):
print news
def startClock(self):
bus.write_byte(self.DS1307_I2C_ADDRESS, 0x00)
self.second = bus.read_byte(self.DS1307_I2C_ADDRESS) & 0x7f
bus.write_byte_data(self.DS1307_I2C_ADDRESS, 0x00, self.second)
print 'startClock..'
def stopClock(self):
bus.write_byte(self.DS1307_I2C_ADDRESS, 0x00)
self.second = bus.read_byte(self.DS1307_I2C_ADDRESS) | 0x80
bus.write_byte_data(self.DS1307_I2C_ADDRESS, 0x00, self.second)
print 'stopClock..'
def setTime(self):
data = [self.decToBcd(self.second), self.decToBcd(self.minute), \
self.decToBcd(self.hour), self.decToBcd(self.dayOfWeek), \
self.decToBcd(self.dayOfMonth), self.decToBcd(self.month), \
self.decToBcd(self.year)]
bus.write_byte(self.DS1307_I2C_ADDRESS, 0x00)
bus.write_i2c_block_data(self.DS1307_I2C_ADDRESS,0x00,data)
print 'setTime..'
def getTime(self):
bus.write_byte(self.DS1307_I2C_ADDRESS, 0x00)
data = bus.read_i2c_block_data(self.DS1307_I2C_ADDRESS,0x00)
#A few of these need masks because certain bits are control bits
self.second = self.bcdToDec(data[0] & 0x7f)
self.minute = self.bcdToDec(data[1])
self.hour = self.bcdToDec(data[2] & 0x3f) #Need to change this if 12 hour am/pm
self.dayOfWeek = self.bcdToDec(data[3])
self.dayOfMonth = self.bcdToDec(data[4])
self.month = self.bcdToDec(data[5])
self.year = self.bcdToDec(data[6])
print 'getTime..'
def fillByHMS(self, _hour, _minute, _second):
self.hour = _hour
self.minute = _minute
self.second = _second
print 'fillByHMS..'
def fillByYMD(self, _year, _month, _day):
self.year = _year - 2000
self.month = _month;
self.dayOfMonth = _day
print 'fillByYMD..'
def fillDayOfWeek(self, _dow):
self.dayOfWeek = _dow
print 'fillDayOfWeek..'
if __name__ == "__main__":
clock = DS1307()
clock.fillByYMD(2015,3,5)
clock.fillByHMS(12,42,30)
clock.fillDayOfWeek(clock.THU)
clock.setTime()
while True:
clock.getTime()
print clock.hour, ":", clock.minute, ":", \
clock.second, " ", clock.dayOfMonth, "/", \
clock.month, "/", clock.year," ", "weekday", \
":", clock.dayOfWeek
time.sleep(1)
Excute below commands to use this sensor
sudo python grove_rtc.py
Here is the result.
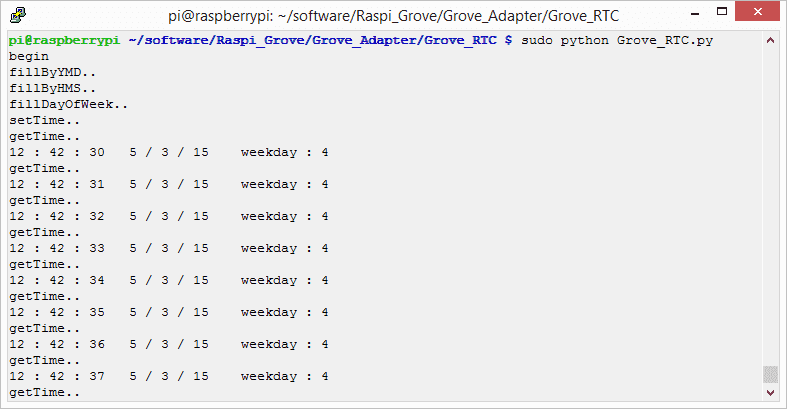
Schematic Online Viewer
Resources
- [Eagle] Grove-RTC in Eagle format
- [PDF] Grove-RTC Schematic in PDF format
- [PDF] Grove-RTC PCB in PDF format
- [Library]Github repository for RTC
- [Datasheet] DS1307 Datasheet
Projects
Using a Real Time Clock With Arduino: Ever wanted to make a project that activated on a schedule? Using a Real Time Clock (RTC) is a great way to do it!
Colorful Clock: A clock that shows your energy level and greets you when you approach
Tech Support & Product Discussion
Thank you for choosing our products! We are here to provide you with different support to ensure that your experience with our products is as smooth as possible. We offer several communication channels to cater to different preferences and needs.