Face Detection with reTerminal and Pi camera with OpenCV
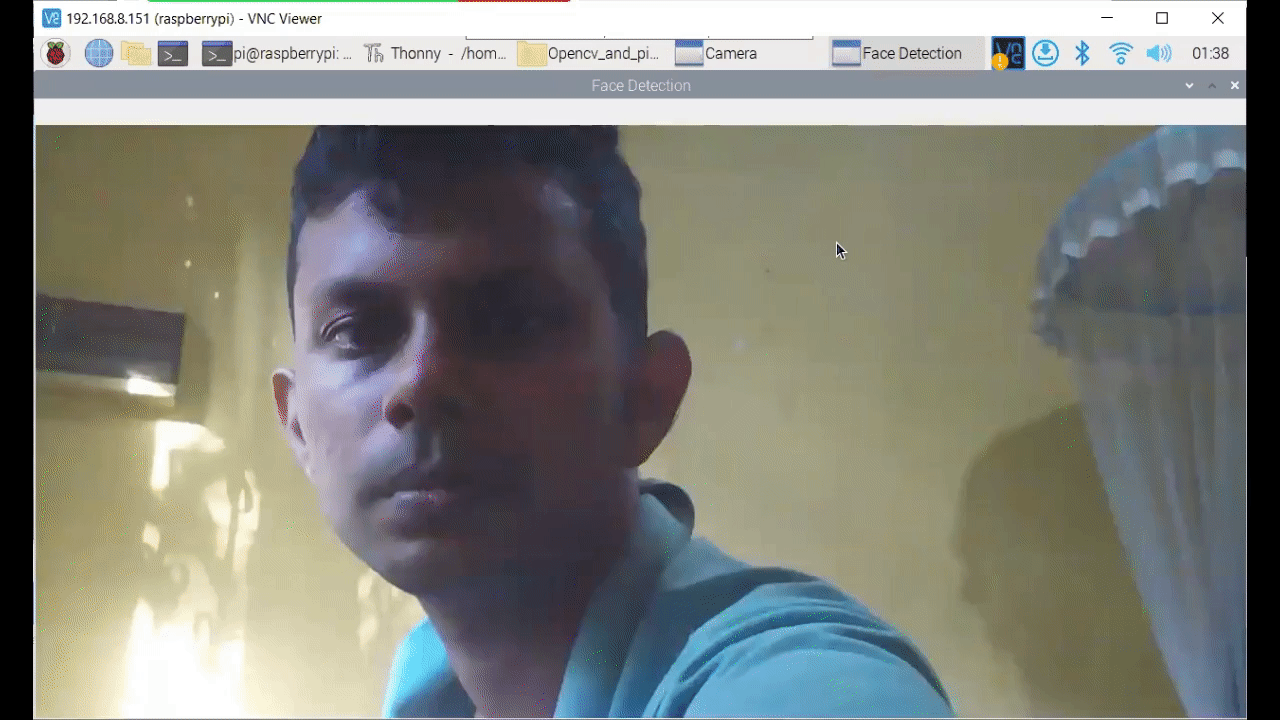
Introduction
Face detection using the Haar Cascade method is a pivotal technique in computer vision, employing a machine learning model to recognize facial features. This method relies on a cascade of classifiers trained on Haar-like features, allowing for swift and accurate identification of faces in images and video streams. Its widespread application spans diverse fields, including facial recognition technology for security and authentication purposes, video surveillance systems, and even in photography software for automatic tagging and sorting based on detected faces. The Haar Cascade method is particularly valuable due to its computational efficiency, making it well-suited for real-time face detection in various contexts, contributing significantly to the advancement of facial analysis and identification technologies.
Getting Start
Before you start this project, you may need to prepare your hardware and software in advance as described here.
Hardware preparation
reTerminal | PiCam |
---|---|
![]() | ![]() |
Software Preparation
We recommend installing the Bullesye or Bullseye version of Raspberry Pi 64 bit OS from their official website. If you prefer to install a new Raspbian OS, please follow the steps outlined in this guide.
We highly recommend checking out our previous tutorial on Getting started with OpenCV, as this tutorial serves as a continuation in our series.
What is Haar Cascade method?
In OpenCV, the Haar Cascade method is a face detection algorithm based on machine learning. It works by training a cascade classifier on positive and negative images, allowing it to recognize patterns and features indicative of faces. The cascade comprises multiple stages, each with a set of weak classifiers that progressively eliminate non-face regions, making the detection process efficient. Haar-like features, resembling rectangular patterns of dark and light areas, serve as the foundation for recognizing objects, such as faces. Once trained, the cascade can be applied to images or video frames to detect faces quickly. This method is widely used for its accuracy and speed, making it a popular choice in various applications, including real-time face detection and recognition.
Please go though with this documentation if you want to learn more.
Let's run the code.
Make sure that you are in correct folder. If not
cd Seeed_Python_ReTerminal/samples/Opencv_and_piCam
Then or Even you can use Thonny IDE to run the python script.
python facedetection_pi.py
Following your reference, we'll provide the entire code snippet for your convenience.
import cv2
from picamera2 import Picamera2
# Load the pre-trained Haar Cascade classifier for face detection
face_cascade = cv2.CascadeClassifier(cv2.data.haarcascades + 'haarcascade_frontalface_default.xml')
picam2 = Picamera2()
picam2.preview_configuration.main.size = (1280, 720)
picam2.preview_configuration.main.format = "RGB888"
picam2.preview_configuration.align()
picam2.configure("preview")
picam2.start()
try:
while True:
im = picam2.capture_array()
cv2.imshow("Camera", im)
# Convert the image to grayscale for face detection
gray = cv2.cvtColor(im, cv2.COLOR_BGR2GRAY)
# Perform face detection
faces = face_cascade.detectMultiScale(gray, scaleFactor=1.3, minNeighbors=5, minSize=(30, 30))
# Draw rectangles around the detected faces
for (x, y, w, h) in faces:
cv2.rectangle(im, (x, y), (x+w, y+h), (255, 0, 0), 2)
# Display the image with face detection
cv2.imshow("Face Detection", im)
# Break the loop when 'q' is pressed
if cv2.waitKey(1) == ord('q'):
break
finally:
# Release resources
cv2.destroyAllWindows()
picam2.stop()
picam2.close()
If you want to find more haas cascades xml files to play around, please go through with this link.
Applications
Haarcascade, originally designed for real-time face detection, has undergone advancements to address contemporary challenges. Innovators have extended its capabilities to include face mask detection, crucial for public health measures. Furthermore, the technology has been optimized for license plate detection, particularly valuable in low-resource environments where sophisticated hardware is scarce. These adaptations demonstrate Haarcascade's versatility and efficiency in catering to diverse needs within computer vision applications.
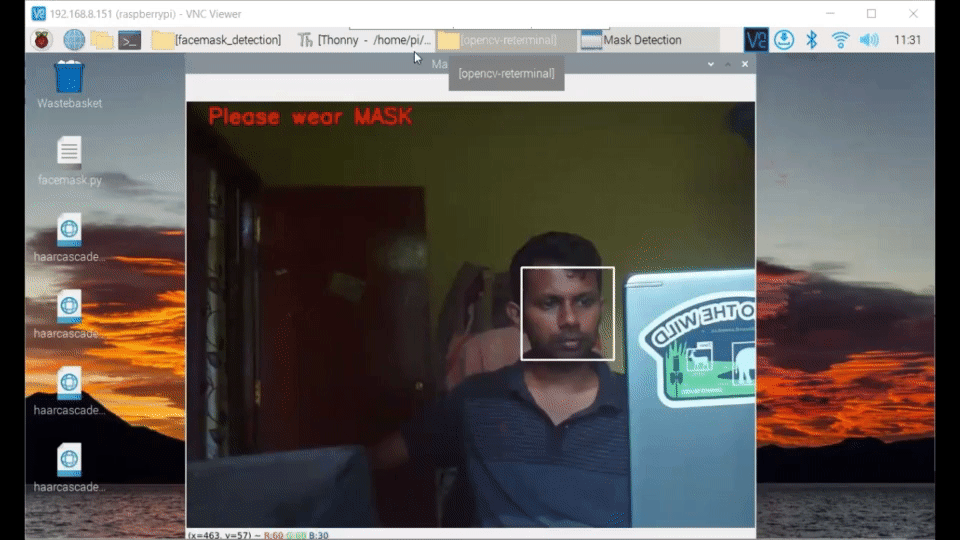
Tech support
Thank you for choosing our products! We are here to provide you with different support to ensure that your experience with our products is as smooth as possible. We offer several communication channels to cater to different preferences and needs.