Seeed Studio XIAO SAMD21 with MicroPython
Introduction of MicroPython
MicroPython is a Python interprer with a partial native code compilation feature. It provides a subset of Python 3.5 features, implemented for embedded processors and constrained systems. It is different from CPython and you can read more about the differences here.
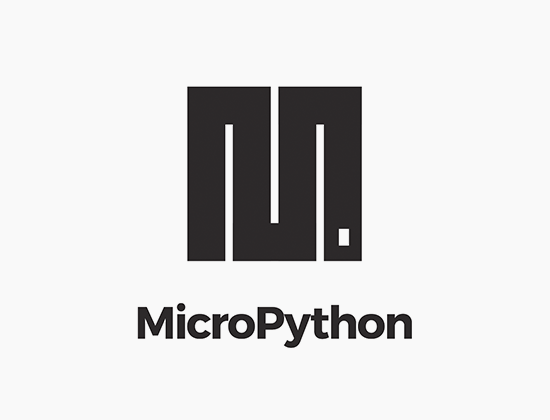
Getting Started
First, we are going to connect the Seeed Studio XIAO SAMD21 to the computer and upload a simple code from MicroPython to check whether the board is functioning well.
Hardware Setup
- Seeed Studio XIAO SAMD21 x1
- Type-C cable x1
- PC x1
Software Setup
- Step 1. Download and Install the latest version of Thonny editor according to your operating system
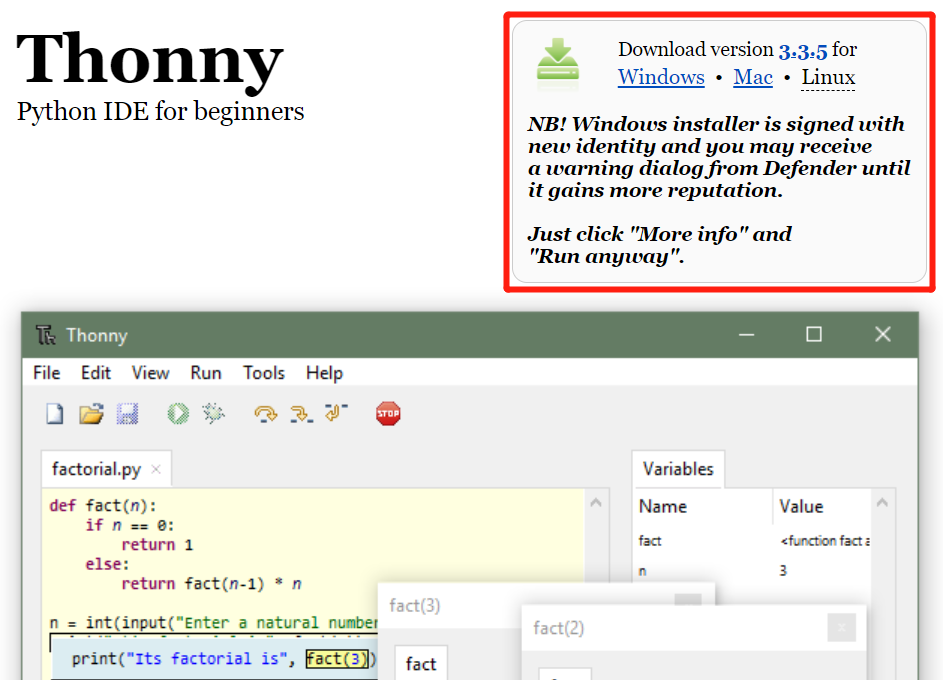
Step 2. Launch the Thonny
Step 3. Click "Tools-->Options" to open the settings.
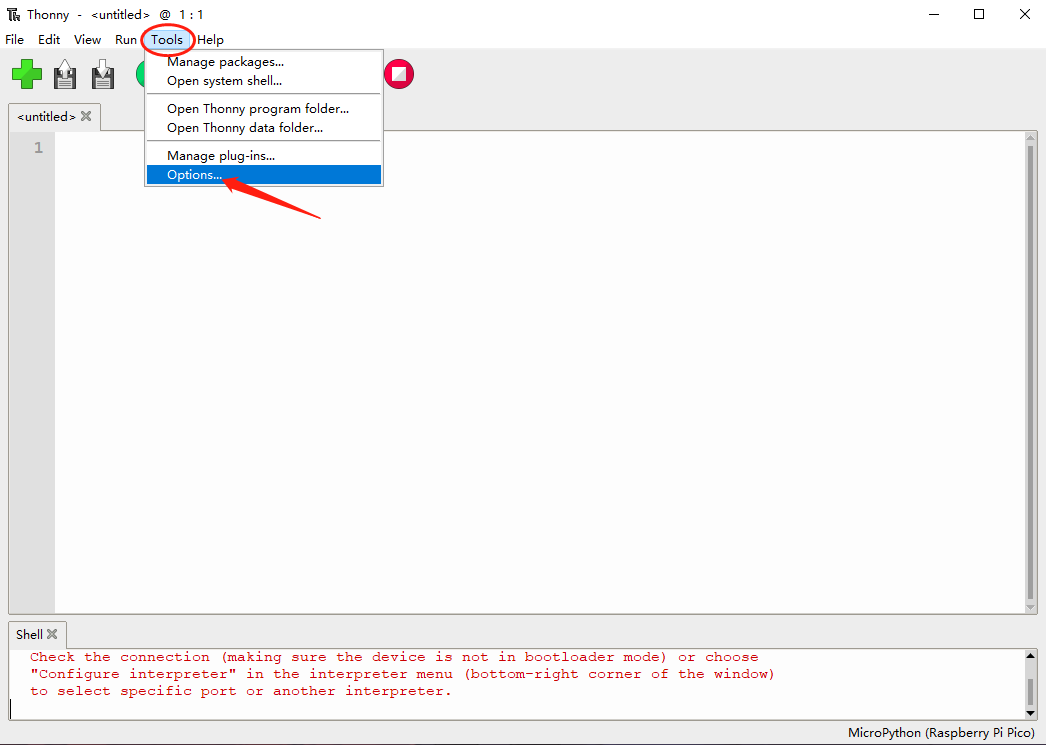
- Step 4. Chose the "Interpreter" interface and select the device as "MicroPython(generic)" and the port as "Try to detect prot automatically"
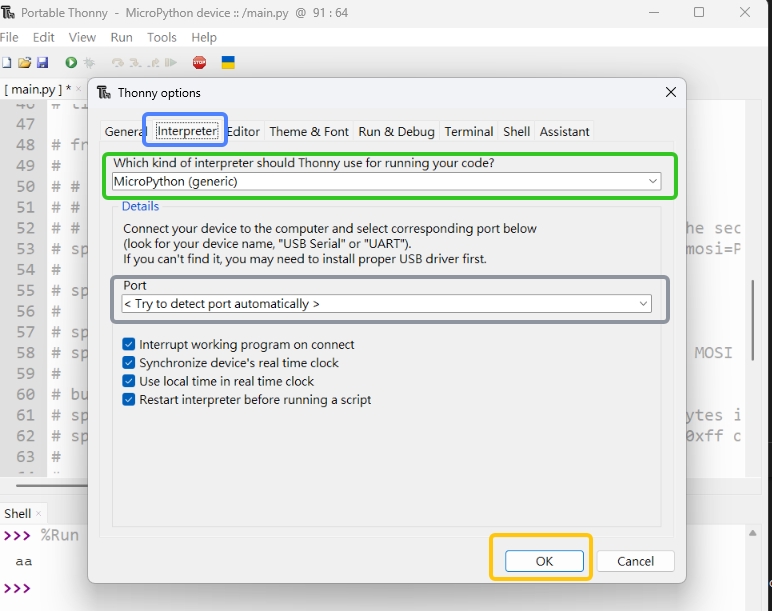
Connect Seeed Studio XIAO SAMD21 to the PC and Light it up
- Step 1. Press and hold the "BOOT" button and then connect the Seeed Studio XIAO SAMD21 to the PC through the Type-C cable. If it works well, there is an "Arduino" desk shown on the PC.
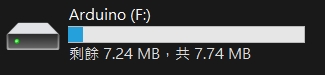
- Step 2. Flash the firmware
For windows:
Copy the Seeed XIAO SAMD21 firmware for MicroPython Support and place it in this folder
For Linux:
wget "https://micropython.org/resources/firmware/SEEED_XIAO-20220618-v1.19.1.uf2"
cp SEEED_XIAO-20220618-v1.19.1.uf2 /media/$USER/Arduino/
It is also possible to compile your own firmware in order to ensure security and support for the latest features, but this is not necessary.
Software Development
SEEED XIAO SAMD21 pin assignment table
Pin | GPIO | Xiao Pin name | IRQ | ADC |
---|---|---|---|---|
2 | PA02 | 0 | 2 | 0 |
4 | PA04 | 1 | 4 | 4 |
10 | PA10 | 2 | 10 | 18 |
11 | PA11 | 3 | 11 | 19 |
8 | PA08 | 4 | * | 16 |
9 | PA09 | 5 | 9 | 17 |
40 | PB082 | 6 | 8 | 2 |
41 | PB09 | 7 | 9 | 3 |
7 | PA07 | 8 | 7 | 7 |
5 | PA05 | 9 | 5 | 5 |
6 | PA06 | 10 | 6 | 6 |
18 | PA18 | RX_LED | 2 | * |
30 | PA30 | SWCLK | 10 | * |
31 | PA31 | SWDIO | 11 | * |
19 | PA19 | TX_LED | 3 | * |
Upload your code
Upload the codes by clicking the "Run current script" button. For the first time, Thonny will ask where you want to save your codes file. Both This Computer and MicroPython device are fine.
If you want to use the program offline, you should save the program to XIAO SAMD21
Press and hold Ctrl + Shift + S at the same time , then select save to MicroPython device
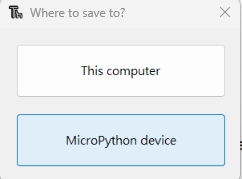
GPIO TEST (LED)
We need to prepare:
Copy the following codes to Thonny.
We can see that the blue RX_LED is lit up and blinks once per second
from machine import Pin, Timer
led = Pin(18, Pin.OUT)
Counter = 0
Fun_Num = 0
def fun(tim):
global Counter
Counter = Counter + 1
print(Counter)
led.value(Counter%2)
tim = Timer(-1)
tim.init(period=500, mode=Timer.PERIODIC, callback=fun)
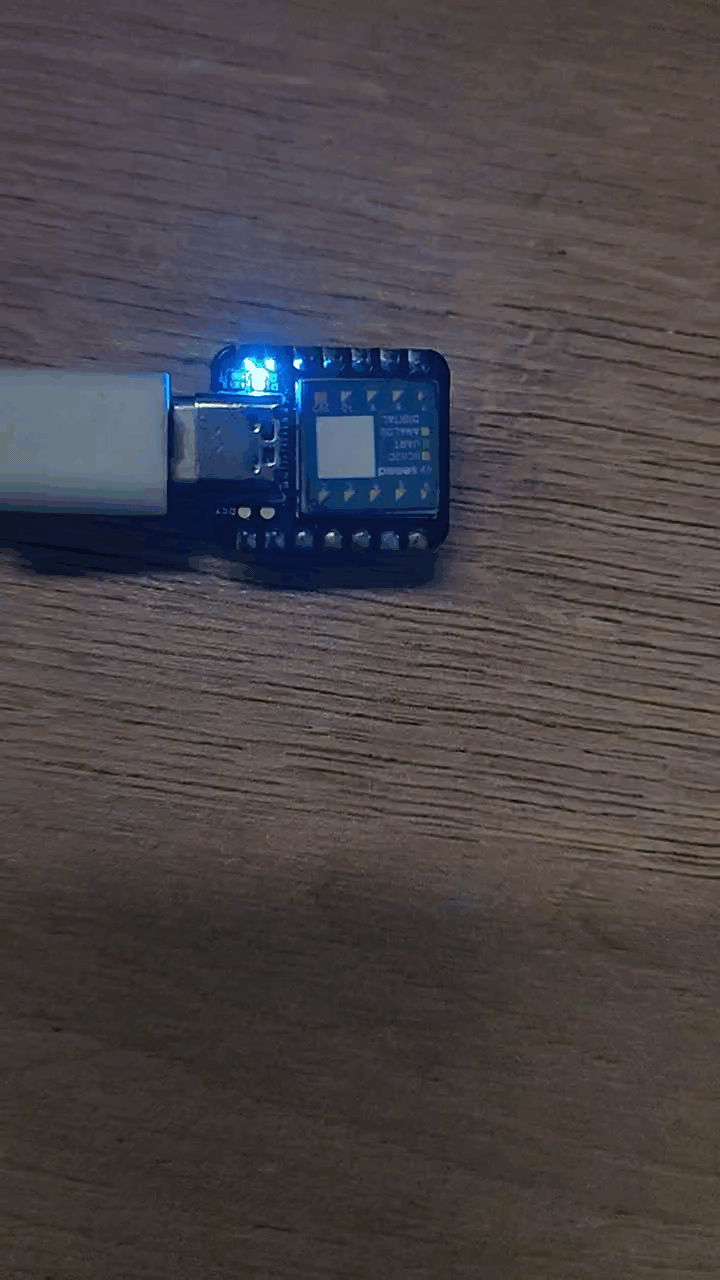
GPIO Control Relays
We need to prepare:
from machine import Pin, Timer
output_4 = Pin(8, Pin.OUT)
detect_1 = Pin(4, Pin.IN, Pin.PULL_UP)
output_value = Pin(2, Pin.OUT)
Counter = 0
def fun(tim):
global Counter
Counter = Counter + 1
output_4.value(Counter%2)
print(Counter%2,detect_1.value())
if detect_1.value() :
output_value.value(1)
else:
output_value.value(0)
tim = Timer(-1)
tim.init(period=200, mode=Timer.PERIODIC, callback=fun)
Human detection for automatic control
We need to prepare:
from machine import Pin, Timer
led = Pin(8, Pin.OUT)
input_value_1 = Pin(4, Pin.IN, Pin.PULL_UP)
input_value_2 = Pin(10, Pin.IN, Pin.PULL_UP)
output_value = Pin(2, Pin.OUT)
Counter = 0
Fun_Num = 0
def fun(tim):
global Counter
Counter = Counter + 1
led.value(Counter%2)
print(input_value_1.value(),input_value_2.value())
if input_value_1.value() :
output_value.value(1)
else:
output_value.value(0)
tim = Timer(-1)
tim.init(period=50, mode=Timer.PERIODIC, callback=fun)
I2C Support
from machine import Pin, SoftI2C
i2c = SoftI2C(scl=Pin(9), sda=Pin(8), freq=100000)
devices = i2c.scan()
for device in devices:
print("Decimal address: ",device," | Hexa address: ",hex(device))
i2c.writeto(0x51, 'b')
print(i2c.readfrom(0x51, 4)) # read 4 bytes from device with address 0x51
i2c.writeto(0x51, 'a') # write 'a' to device with address 0x51
print(i2c.readfrom(0x51, 4)) # read 4 bytes from device with address 0x51
i2c.writeto(0x51, 'b')
print(i2c.readfrom(0x51, 4))
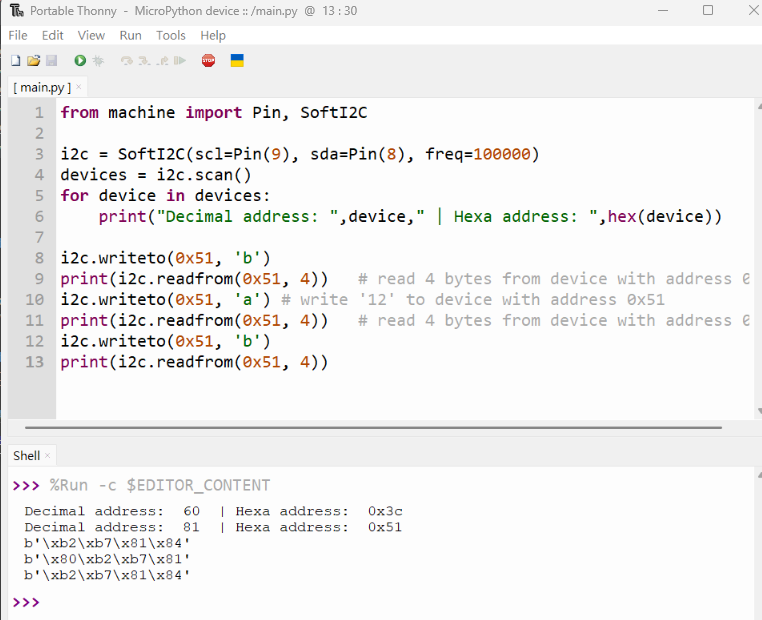
Familiarity with micropython allows you to do more , we are looking forward to creating more value for you. Feel free to share your projects with us too!
MicroPython Device Console
Our partner Neil has written a command line console program for XIAO using MicroPython. With this programme you can easily upload, download and delete files. We thank him for his contribution to XIAO!
Tech Support & Product Discussion
Thank you for choosing our products! We are here to provide you with different support to ensure that your experience with our products is as smooth as possible. We offer several communication channels to cater to different preferences and needs.